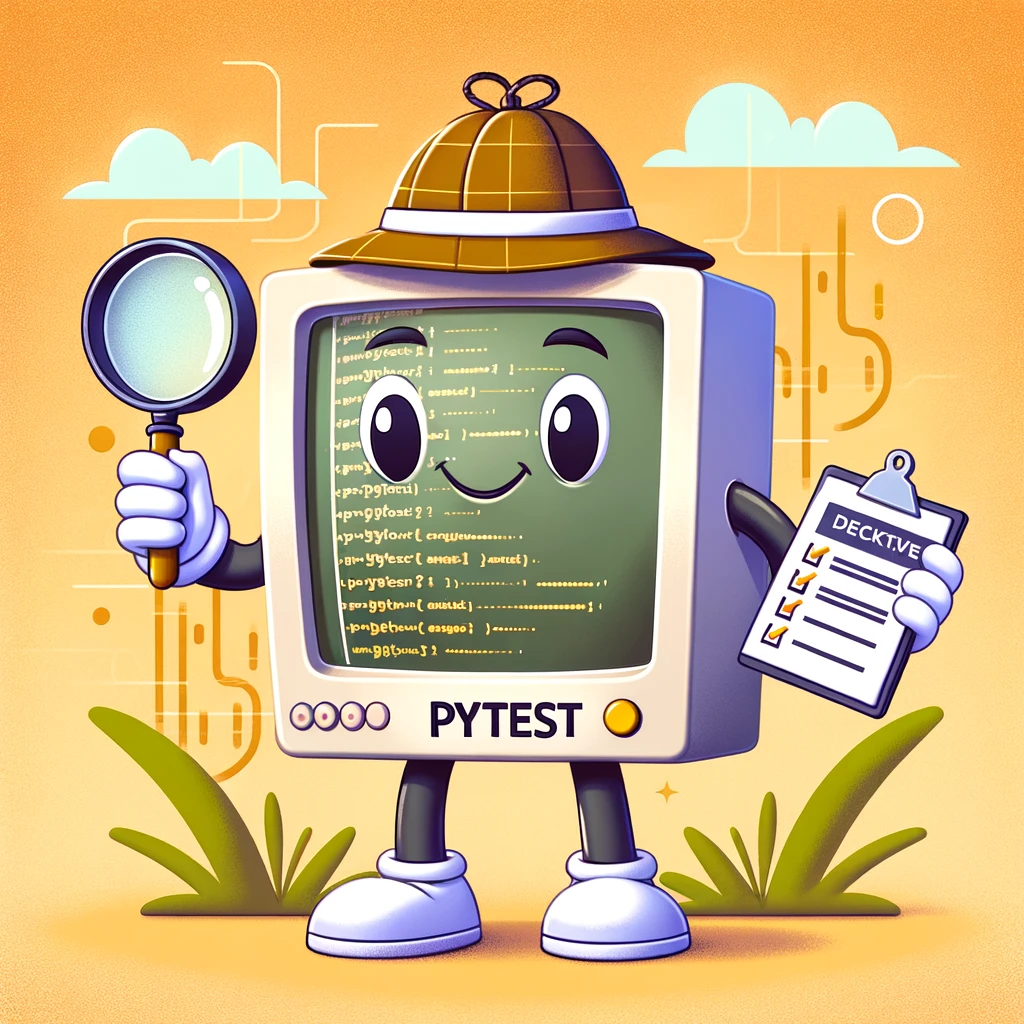
Testing
Testing is an essential part of software development that ensures your code works as expected and helps maintain its reliability over time.
Pytest is a powerful, no-boilerplate-needed testing framework in Python that makes writing simple and scalable test cases easy. Let’s explore how you can leverage Pytest in your Python projects.
Why Pytest?
Pytest is a popular testing framework for Python due to its simplicity and flexibility. It supports powerful fixtures, has a rich plugin architecture, and can easily integrate with other testing tools and frameworks.
With Pytest, writing tests becomes more pythonic and less cumbersome compared to other frameworks like unittest.
Setting Up Pytest
First, you need to install Pytest. Simply run:
pip install pytest
After installation, you can start writing your test cases.
Example Project Structure
Imagine you have a simple Python project with the following structure:
my_project/
app.py
tests/
test_app.py
In app.py
, you have a function you want to test. For instance:
# app.py
def add_numbers(a, b):
"""Add two numbers."""
return a + b
Writing Your First Test
Now, let’s write a test for the add_numbers
function in test_app.py
.
# tests/test_app.py
from app import add_numbers
def test_add_numbers():
assert add_numbers(2, 3) == 5
This test checks if the add_numbers
function correctly adds two numbers.
Running the Tests
To run your tests, simply execute:
pytest
Pytest will automatically discover and run all tests in the tests
directory.
Parametrizing Tests
One of the powerful features of Pytest is parameterized tests. This allows you to run the same test function with different inputs. For example:
# tests/test_app.py
import pytest
from app import add_numbers
@pytest.mark.parametrize("a, b, expected", [(2, 3, 5), (4, 5, 9), (0, 0, 0)])
def test_add_numbers_parametrized(a, b, expected):
assert add_numbers(a, b) == expected
This will run test_add_numbers_parametrized
three times with different sets of arguments.
Handling Exceptions
Testing exceptions is straightforward with Pytest. Suppose you have a function that raises an exception:
# app.py
def divide_numbers(a, b):
if b == 0:
raise ValueError("Cannot divide by zero")
return a / b
You can write a test to ensure the exception is raised correctly:
# tests/test_app.py
import pytest
from app import divide_numbers
def test_divide_numbers_exception():
with pytest.raises(ValueError):
divide_numbers(10, 0)
Conclusion
Testing with Pytest is a vital part of the Python development process. It’s straightforward, flexible, and powerful. By integrating testing into your workflow, you can write more reliable and maintainable code. Remember, a well-tested application is a robust application!
Other Reading
4 Techniques for Testing Python Command-Line (CLI) Apps – Real Python
Get Started — pytest documentation
Pytest – Starting With Basic Test
Testing Python Applications with Pytest – Semaphore Tutorial